일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
Tags
- API플랫폼
- Endpoint
- 웹크롤링
- udemy
- 프로그램
- 파이썬
- 게임
- Game
- 부트스트랩
- 웹페이지
- 파싱
- ndarray
- HTTP
- class
- 유데미
- phython
- 오류
- 쉬티
- 상태코드
- API
- SMTP
- 프로젝트
- 최저가
- twilio
- HTML
- Python
- Pygame
- Sheety
- Tequila
- 계산기
Archives
- Today
- Total
데이터 분석가
PDF파일 음성 변환 Pdf2Voice (Udemy Project 9) 본문
안녕하세요!
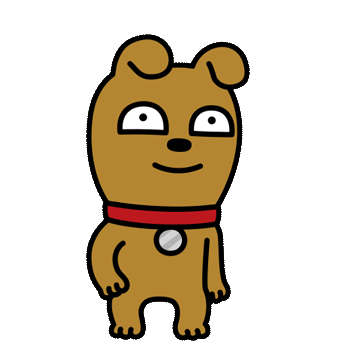
이번 시간에는 PDF 파일을 읽어 음성으로 변환시키는 PDF 리더기 파이썬 코드를 구축해보겠습니다 !
작동 원리는
Flask 웹 프레임워크를 사용하여 PDF 파일을 업로드하고
업로드한 파일에서 텍스트를 추출한 후 이를 음성으로 변환하여
다운로드할 수 있는 웹 애플리케이션입니다
구성
1.upload.html
2.play.html
3.PDF2Voice.py
으로 이루어져 있고
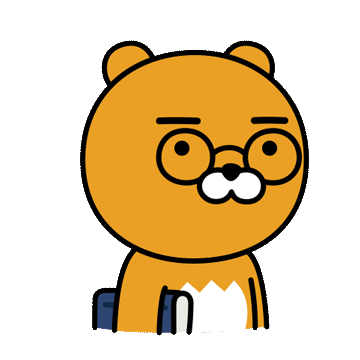
1.upload.html
<!DOCTYPE html>
<html>
<head>
<title>파일 업로드</title>
<style>
body {
margin: 0;
padding: 0;
overflow: hidden;
background: url(C:\Users\hospc\Desktop\Final_Project\no.9 PDF\stars.mp4) no-repeat center center fixed;
-webkit-background-size: cover;
-moz-background-size: cover;
-o-background-size: cover;
background-size: cover;
}
#content {
position: relative;
z-index: 1;
width: 100%;
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
font-family: Arial, sans-serif;
color: #ffffff;
text-align: center;
}
.container {
width: 400px;
padding: 20px;
background-color: rgba(0, 0, 0, 0.7);
border-radius: 10px;
}
.container h1 {
margin-bottom: 20px;
}
.container ul.messages {
list-style: none;
padding: 0;
margin-bottom: 20px;
}
.container ul.messages li {
margin-bottom: 5px;
}
.container form {
text-align: center;
}
.container input[type="file"] {
margin-bottom: 10px;
}
.container input[type="submit"] {
background-color: #4caf50;
color: #ffffff;
border: none;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
border-radius: 5px;
cursor: pointer;
}
</style>
</head>
<body>
<div id="content">
<div class="container">
<h1>파일 업로드</h1>
{% with messages = get_flashed_messages() %}
{% if messages %}
<ul class="messages">
{% for message in messages %}
<li>{{ message }}</li>
{% endfor %}
</ul>
{% endif %}
{% endwith %}
<form method="post" enctype="multipart/form-data" action="{{ url_for('upload') }}">
<input type="file" name="file" accept=".pdf">
<input type="submit" value="업로드">
</form>
</div>
</div>
</body>
</html>
2.play.html
<!DOCTYPE html>
<html>
<head>
<title>음성 재생</title>
</head>
<body>
<h1>음성 재생</h1>
<audio controls autoplay>
<source src="{{ url_for('static', filename=filename) }}" type="audio/mpeg">
</audio>
<p><a href="{{ url_for('upload') }}">새로운 파일 업로드</a></p>
</body>
</html>
결과
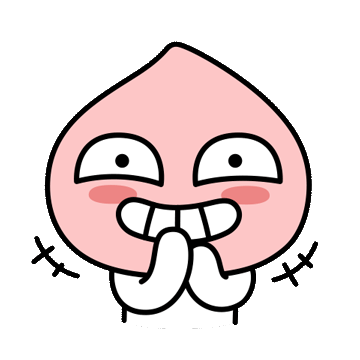
3.PDF2Voice.py
from flask import Flask, render_template, request, flash, redirect, url_for, send_file
import os
import tempfile
import pyttsx3
from PyPDF2 import PdfReader
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your_actual_secret_key_here'
ALLOWED_EXTENSIONS = {'pdf'}
def allowed_file(filename):
# 파일 확장자가 허용된 확장자인지 확인합니다
return '.' in filename and filename.rsplit('.', 1)[1].lower() in ALLOWED_EXTENSIONS
def extract_text_from_pdf(file_path):
# PDF 파일로부터 텍스트 내용을 추출합니다
with open(file_path, 'rb') as file:
pdf_reader = PdfReader(file)
text = ''
for page in pdf_reader.pages:
text += page.extract_text()
return text
def convert_text_to_speech(text):
# pyttsx3 라이브러리를 사용하여 텍스트를 음성으로 변환합니다
engine = pyttsx3.init()
output_dir = 'C:\\Users\\hospc\\Desktop\\Final_Project\\no.9 PDF'
os.makedirs(output_dir, exist_ok=True) # 출력 디렉토리가 없으면 생성합니다
save_path = os.path.join(output_dir, 'output.mp3') # 출력 오디오 파일의 경로를 지정합니다
engine.save_to_file(text, save_path)
engine.runAndWait()
return save_path
@app.route('/', methods=['GET', 'POST'])
def upload():
if request.method == 'POST':
if 'file' not in request.files:
flash('파일이 없습니다.')
return redirect(request.url)
file = request.files['file']
if file.filename == '':
flash('파일이 선택되지 않았습니다.')
return redirect(request.url)
if not allowed_file(file.filename):
flash('PDF 파일만 업로드할 수 있습니다.')
return redirect(request.url)
temp_dir = tempfile.mkdtemp(dir='C:\\Users\\hospc\\Desktop\\Final_Project')
file_path = os.path.join(temp_dir, file.filename)
file.save(file_path)
text = extract_text_from_pdf(file_path)
if text:
save_path = convert_text_to_speech(text)
flash('음성 변환이 완료되었습니다.')
return redirect(url_for('download', filename=os.path.basename(save_path)))
else:
flash('PDF 파일에서 텍스트를 추출할 수 없습니다.')
return redirect(request.url)
return render_template('upload.html')
@app.route('/download/<filename>')
def download(filename):
filepath = os.path.join('C:\\Users\\hospc\\Desktop\\Final_Project\\no.9 PDF', filename)
if os.path.exists(filepath) and os.path.isfile(filepath):
return send_file(filepath, as_attachment=True)
else:
flash('파일을 찾을 수 없습니다.')
return redirect(url_for('upload'))
if __name__ == '__main__':
app.run(debug=True)
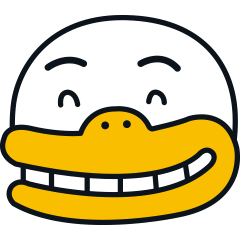
저는 코드들의 작동 방식은
Flask 웹 프레임워크를 사용하여 PDF 파일을 업로드하고
업로드한 파일에서 텍스트를 추출한 후 이를 음성으로 변환하여
다운로드할 수 있는 웹 애플리케이션입니다
잘 됩니다 !!
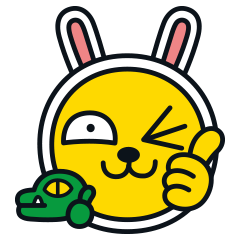
'유데미 부트캠프 프로젝트(Final)' 카테고리의 다른 글
MLB 선수들 데이터 스크래핑(Udemy Project 11) (0) | 2023.07.01 |
---|---|
이미지 파일 색상 분석 (Udemy Project 10) (0) | 2023.06.30 |
파이썬 가장 위험한 글쓰기 (udemy project 8) (0) | 2023.06.28 |
ToDoList 웹 페이지 구축 (Udemy Project 7) (0) | 2023.06.28 |
노트북 하기 좋은 카페 찾기 프로젝트(Udemy Final Project 6) (0) | 2023.06.27 |
Comments