일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
- 상태코드
- 파이썬
- 쉬티
- Sheety
- 유데미
- twilio
- 부트스트랩
- ndarray
- 웹페이지
- Pygame
- Endpoint
- 최저가
- Game
- API
- 프로그램
- 프로젝트
- Python
- SMTP
- 파싱
- 웹크롤링
- 게임
- 오류
- udemy
- phython
- Tequila
- HTTP
- class
- HTML
- API플랫폼
- 계산기
- Today
- Total
데이터 분석가
파이썬(Python) 블랙잭(Blackjack) 프로젝트 본문
안녕하세요 !!
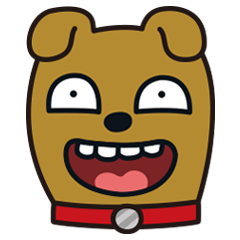
이번 시간에는 블랙잭을 만드는 코드를 짜 볼 건데요
이 카드 게임에 대해 간단히 설명하자면, 카드를 뽑아 21에 가깝거나 21을 만들면 이기는 게임입니다 !
import random
from replit import clear
from art import logo
def deal_card():
"""덱에서 임의의 카드를 사용합니다"""
cards = [11, 2, 3, 4, 5, 6, 7, 8, 9, 10, 10, 10, 10] #11은 클로버 에이스 카드
card = random.choice(cards) #card는 cards 목록에서 랜덤 뽑기
return card #랜덤으로 뽑힌 카드를 리턴 반환
가장 먼저 첫 번째 def deal_card(): 라는 cards 리스트 내에 있는 카드를 랜덤 선택하여
그 값을 리턴하는 def문을 선언합니다.
def calculate_score(cards):
"""뽑은 카드를 계산한다"""
if sum(cards) == 21 and len(cards) == 2: #11과 10을 뽑았을 경우이다
return 0 #0을 반환
if 11 in cards and sum(cards) > 21: #21을 넘었는데 클로버 에이스(11)을 가질 경우
cards.remove(11) #에이스(1,11) 둘 중 선택 가능하기에
cards.append(1) #1로 대체한다
return sum(cards) #1로 대체한 값을 다시 계산한다
첫 번째 def deal_card(): 을 통해 선택된 카드 값들을
두 번째 def calculate_score(cards) 를 이용해 값들을 합산하거나, 블랙잭(21)일 경우 0, 에이스(1 or 11)를 가질 경우
를 계산합니다. 이 때 , 에이스를 11로 계산하였는데 21을 넘기는 버스트일 경우 1로 변환.
def compare(user_score, computer_score): #유저와 컴퓨터를 비교하는 함수
if user_score > 21 and computer_score > 21: #유저 21 초과, 컴퓨터 21 초과일 경우
return "You went over. You lose .·´¯`(>▂<)´¯`·. " #당신은 졌습니다
if user_score == computer_score: #비길 경우
return "Draw ╚(•⌂•)╝" #무승부
elif computer_score == 0: #컴퓨터가 블랙잭 21(0은 블랙잭이다)일 경우
return "Lose, Opponent has Blackjack (●'◡'●)" #컴퓨터는 블랙잭이다
elif user_score == 0: #유저가 블랙잭일 경우
return "Win with a Blackjack ☆*: .。. o(≧▽≦)o .。.:*☆" # 이겼다
elif user_score > 21: #유저가 버스트(21초과)일 경우
return "You went over. You lose ༼ つ ◕_◕ ༽つ" #당신은 졌습니다
elif computer_score > 21: #컴퓨터 버스트(21초과)
return "Opponent went over. You Win (●'◡'●)" #상대방은 버스트로 당신이 이겼습니다
elif user_score > computer_score: #유저가 컴퓨터보다 21에 가까운 수
return "You Win 👍😁"
else:
return "You Lose 🤣" #컴퓨터 보다 숫자가 작은 수 이므로 패배
세 번째 def compare(user_score, computer_score) 을 이용해서 사용자와, 컴퓨터를 비교합니다.
각각의 경우의 수에 대해서 Blackjack 룰대로 표현하여 출력 값을 정합니다.
이 경우 elif를 이용했는데 단순히 사용자와 컴퓨터 중 21을 가지거나 21에 근접한 수를 가진 쪽이 이기게 됩니다
def play_game(): #게임을 시작합니다
print(logo) #로고 출력
user_cards = [] #유저 카드를 여기 리스트에 저장
computer_cards = [] #컴퓨터 카드를 여기 리스트에 저장
is_game_over = False #while not문을 사용하기 전에 False 선언
for _ in range(2): #카드를 2번 뽑는다
user_cards.append(deal_card()) #랜덤 초이스 된 카드를 user_card 리스트 저장
computer_cards.append(deal_card()) #랜덤 초이스 된 카드를 computer_card 리스트
네 번째 def play_game(): 반복문은 사용자가 뽑게 되는 카드를 user_cards = [] 리스트에 저장,
컴퓨터가 뽑게 되는 카드를 computer_cards = [] 리스트에 저장하고
while not 문을 이용하기 전에 Flase 값을 선언합니다.
그 다음 def play_game(): 의 이중 for문은 첫 번째 def deal_card()를 두번 수행하여 리스트에 넣습니다.
while not is_game_over: #False일 경우 게임 중단
# 유저와 컴퓨터 중 블랙잭이거나 21을 넘는 경우 게임 중단
user_score = calculate_score(user_cards) #유저의 뽑은 카드를 더한 값
computer_score = calculate_score(computer_cards) #컴퓨터의 뽑은 카드 더한 값
print(f" Your cards: {user_cards}, current score: {user_score}")
print(f" Computer's first card: {computer_cards[0]}")
if user_score == 0 or computer_score == 0 or user_score > 21: #블랙잭 or 버스트
is_game_over = True
else:
user_should_deal = input("Type 'y' to get another card, type 'n' to pass: ")
if user_should_deal == "y": #"예" 카드를 추가로 더 받는다
user_cards.append(deal_card()) #추가로 더 받은 카드를 리스트에 추가한다
else:
is_game_over = True #"아니오" 카드를 추가로 안 받을 경우
그 다음 다섯 번째 while not 문을 이용하여 이중 for문의 카드 두 번을 뽑은 리스트를 합산하여 user_score, computer_score을
구합니다. 그 결과 값이 0일 경우(블랙잭 = 0) 21이 넘는 버스트(파산)일 경우 True를 이용해 while문을 벗어납니다
else로 해당 사항이 없을 경우 카드를 추가로 받으며 반복합니다.
while computer_score != 0 and computer_score < 17:
#컴퓨터 블랙잭이 아니거나 17보다 작을 경우
computer_cards.append(deal_card()) #17보다 작은 경우 추가로 카드를 드로우
computer_score = calculate_score(computer_cards) #추가로 드로우 한 카드를 합산
print(f" Your final hand: {user_cards}, final score: {user_score}")
print(f" Computer's final hand: {computer_cards}, final score: {computer_score}")
print(compare(user_score, computer_score))
while input("Do you want to play a game of Blackjack? Type 'y' or 'n': ") == "y":
#'y' '예'를 선택할 경우 user_score, computer_score 등 리스트 모두 초기화
clear()
play_game()
여섯 번째 while 문을 이용해 카드를 추가로 드로우 하거나 종료합니다.
마지막 while input문은 'y'를 선택할 경우 user_score, computer_score 등 리스트 값이 모두 초기화 되고
play_game으로 다시 게임을 시작하게 됩니다
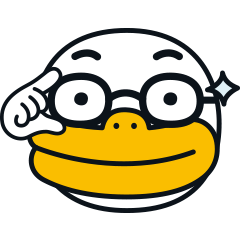
이렇게 우리는 함께 블랙잭 게임에 대해 알아보았는데요, 사실 제가 코드를 짠 블랙잭 게임은
cards = [11, 2, 3, 4, 5, 6, 7, 8, 9, 10, 10, 10, 10] 중에서 계속된 드로우 하는 상황이라
매번 각각의 숫자를 뽑을 확률이 같다는 한계점이 있습니다. . .
아직 제가 많이 부족해서 여기까지가 한계인 것 같습니다..
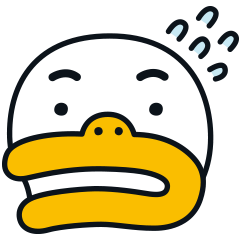
이번 게임은 기존 게임과 비교될 정도로 매우 복잡하게 얽힌 코드였는데요, 그래도 용기내서 한번씩
도전해봅시다 !
'파이썬(python) 프로젝트 모음' 카테고리의 다른 글
파이썬(Python) HigerLower 게임 프로젝트 (0) | 2023.03.24 |
---|---|
파이썬(Python) Up-Down(숫자추측) 게임 프로젝트 (0) | 2023.03.23 |
파이썬(Python) 계산기(calculator) 프로그램 만들기 (0) | 2023.03.22 |
파이썬(Python) 경매(bidding) 프로젝트 (0) | 2023.03.21 |
파이썬(Python) 딕셔너리(dictionary) 채점프로그램, 목록 사전 (0) | 2023.03.21 |